Applications of linked list data structure
A linked list is a linear data structure, in which the elements are not stored at contiguous memory locations. The elements in a linked list are linked using pointers as shown in the below image:
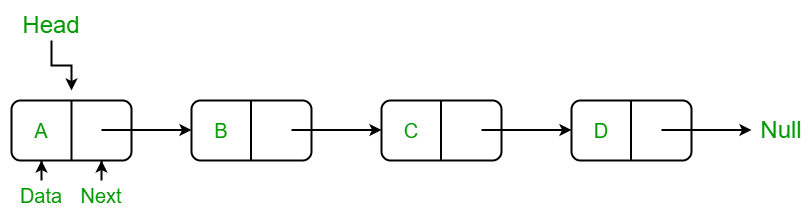
Applications of linked list in computer science:
- Implementation of stacks and queues
- Implementation of graphs: Adjacency list representation of graphs is the most popular which uses a linked list to store adjacent vertices.
- Dynamic memory allocation: We use a linked list of free blocks.
- Maintaining a directory of names
- Performing arithmetic operations on long integers
- Manipulation of polynomials by storing constants in the node of the linked list
- Representing sparse matrices
Applications of linked list in the real world:
- Image viewer – Previous and next images are linked and can be accessed by the next and previous buttons.
- Previous and next page in a web browser – We can access the previous and next URL searched in a web browser by pressing the back and next buttons since they are linked as a linked list.
- Music Player – Songs in the music player are linked to the previous and next songs. So you can play songs either from starting or ending of the list.
- GPS navigation systems- Linked lists can be used to store and manage a list of locations and routes, allowing users to easily navigate to their desired destination.
- Robotics- Linked lists can be used to implement control systems for robots, allowing them to navigate and interact with their environment.
- Task Scheduling- Operating systems use linked lists to manage task scheduling, where each process waiting to be executed is represented as a node in the list.
- Image Processing- Linked lists can be used to represent images, where each pixel is represented as a node in the list.
- File Systems- File systems use linked lists to represent the hierarchical structure of directories, where each directory or file is represented as a node in the list.
- Symbol Table- Compilers use linked lists to build a symbol table, which is a data structure that stores information about identifiers used in a program.
- Undo/Redo Functionality- Many software applications implement undo/redo functionality using linked lists, where each action that can be undone is represented as a node in a doubly linked list.
- Speech Recognition- Speech recognition software uses linked lists to represent the possible phonetic pronunciations of a word, where each possible pronunciation is represented as a node in the list.
- Polynomial Representation- Polynomials can be represented using linked lists, where each term in the polynomial is represented as a node in the list.
- Simulation of Physical Systems- Linked lists can be used to simulate physical systems, where each element in the list represents a discrete point in time and the state of the system at that time.
Applications of Circular Linked Lists:
- Useful for implementation of a queue. Unlike this implementation, we don’t need to maintain two-pointers for the front and rear if we use a circular linked list. We can maintain a pointer to the last inserted node and the front can always be obtained as next of last.
- Circular lists are useful in applications to go around the list repeatedly. For example, when multiple applications are running on a PC, it is common for the operating system to put the running applications on a list and then cycle through them, giving each of them a slice of time to execute, and then making them wait while the CPU is given to another application. It is convenient for the operating system to use a circular list so that when it reaches the end of the list it can cycle around to the front of the list.
- Circular Doubly Linked Lists are used for the implementation of advanced data structures like the Fibonacci Heap.
- Circular linked lists can be used to implement circular queues, which are often used in operating systems for scheduling processes and managing memory allocation.
- Used in database systems to implement linked data structures, such as B+ trees, which are used to optimize the storage and retrieval of data.
- Circular linked lists can be used in networking. For instance, to implement circular buffers for streaming data, such as video and audio, in networking applications.
- Video games use circular linked lists to manage sprite animations. Each frame of the animation is represented as a node in the list, and the last frame is connected to the first frame to create a loop.
- Circular linked lists can be used to represent a buffer of audio or signal data in signal processing applications. The last node is connected to the first node to create a loop, and the processing algorithms can efficiently iterate over the data.
- Traffic light control systems use circular linked lists to manage the traffic light cycles. Each phase of the traffic light cycle is represented as a node in the list, and the last node is connected to the first node to create a loop.
Application of Doubly Linked Lists:
- Redo and undo functionality.
- Use of the Back and forward button in a browser.
- The most recently used section is represented by the Doubly Linked list.
- Other Data structures like Stack, Hash Table, and Binary Tree can also be applied by Doubly Linked List.
- Used to implement game objects and their interactions in a game engine.
- Used in networking.
- Used in Graph algorithms.
- Operating systems use doubly linked lists to manage the process scheduling. Each process waiting to be executed is represented as a node in the doubly linked list, and the operating system can easily traverse the list in both directions to manage the process queue.
Example:
- getMin : Gets minimum
- extractMin : Removes minimum
- getMax : Gets maximum
- extractMax : Removes maximum
- insert : Inserts an item. It may be assumed that the inserted item is always greater than maximum so far. For example, a valid insertion order is 10, 12, 13, 20, 50.